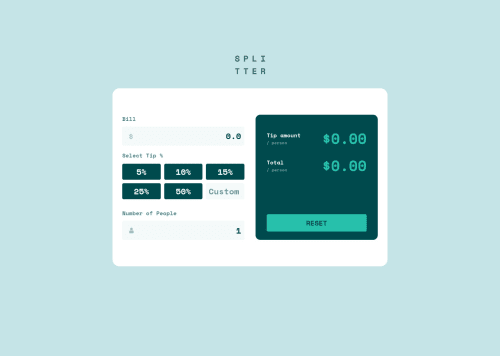
Solution retrospective
Would like to know if there's a way to have input values be valid (not NaN) if the user types his bill value with a comma in between and not a period, also if I enter a bill value and then delete it to enter another the calculator will display NaN on both the tip amount and total and I couldn't find a way to fix it. Any feedback on how to make the layout and functionality better would be much appreciated!
Please log in to post a comment
Log in with GitHubCommunity feedback
- @sedcakmak
Hi Toni,
If you enter a bill value and then delete it, you can get rid of NaN by adding the following code to your calcTip function. This code means: if there is no billValue, set billValue to zero. Your tip amount and total will show zero until you enter a new bill input.
function calcTip() { if(!billValue) billValue = 0; // rest of your code }
Your other question is a little tricky: The
<input type="number">
of your bill input only accepts period as decimal separator, the user cannot even type his bill value with a comma. One workaround I found would be to change your bill input into<input type="text">
and edit your handleBillInput function accordingly:let inputWithComma; function handleBillInput() { if(billInput.value.includes(",")) { inputWithComma= billInput.value.replace(",","."); billValue = parseFloat(inputWithComma) }else { billValue = parseFloat(billInput.value); } calcTip(); }
Another thing i noticed is: you set the values of your billInput and nrOfPeopleInput at your JS file. You can do that on your HTML such as :
<input type="number" id="bill" class="input-field-style" value = "0.0"/>
I hope these will be useful :)
Join our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord