Submitted over 2 years agoA solution to the Tip calculator app challenge
Tip Calculator App with Vanilla JavaScript
accessibility, sass/scss
@kxnzx
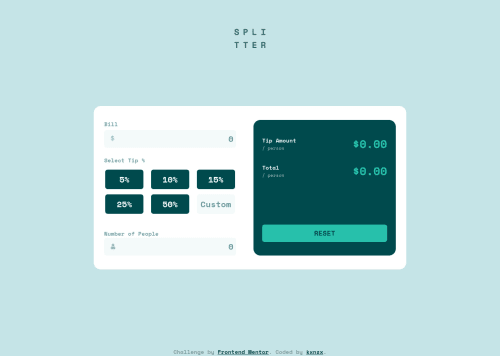
Solution retrospective
Hello Frontend Mentor Gang!
It took me some time to finish this challenge. The calculator works, however the form does not function the way I want it to. Here are some of the issues:
- The error message "Can't be zero" is displayed by default.
- After the reset button has been clicked the value "0" stays set in place after typing in a new value.
My Questions:
- How can I make the error message and the red focus on the input visible only after the tip percentage button or custom input have been filled in?
- How do I make the value "0" dissapear when a new value is typed in after the reset button has been clicked?
Feel free to leave a comment if you've noticed some other issues. Much Appreciated!
Code
Loading...
Please log in to post a comment
Log in with GitHubCommunity feedback
No feedback yet. Be the first to give feedback on kxnzx's solution.
Join our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord